How to Create a weather app using react native
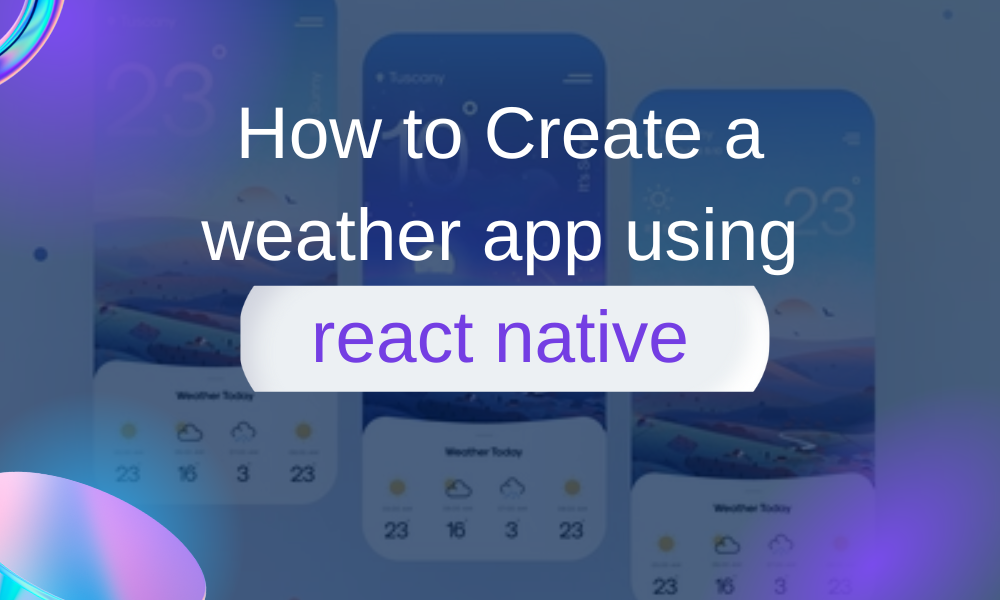
Building an app with React Native provides broad functionality. Not only that you need less coding but you can play with different buttons, components, and React Native hooks. To give a dynamic instance of React Native functionalities, I bring a tutorial blog on ‘Building a weather app using the framework’ that you can try before you hire react native developer. Let’s get along the journey.
Before starting the blog, I want to mention that I have used Expo CLI to create the app. As you know Expo CLI is a tool that simplifies the process of creating a React Native app, this is why the preference.
Not aware of the different types of react native app-making tools?
Now let's start with the first steps toward creating a live weather app. If you are
What are the prerequisite criteria for this project?
- Get a basic idea of the API calling concept.
- Install all the software required to build a React Native project. If you are looking for reliable guidance.
- Learn to build a basic React Native app.
The first step- building an app
In this step, you need to create a basic app and make changes to the loading screen. Let’s see how you can achieve this step.
- Open a command prompt and run npx create-expo-app weather-app. With this, you will see a weather app has been created in your local drive.
- Next, you need to update the splash screen, and loading screen and add some images in the project directory.
- Go to the ./app.js folder on your code editor and change the text element showing <Text>Open up App.js to start working on your app!</Text> to some different texts. Here, I have changed to <Text>Welcome to the Weather forecasting app</Text>. This will give an output like image 1.
Image 1
Usage of isLoading state
Let's discuss the utility of the isLoading state in this project. You will need the boolean isLoading state to specify the initial state of the app. If you set the isLoading state as ‘true’, you tell that the data that your app wants to get is still loading. You can also turn it as false if it's already done with fetching data and not loading. Now let us see an example.
export default class App extends React.Component {
state = {
isLoading: false
};
render() {
const { isLoading } = this.state;
return (
<View style={styles.container}>
{isLoading ? null : (
<View>
<Text>Welcome to the Weather forecasting app.</Text>
</View>
- Here, what I have done is set the isLoading state as false. Simultaneously, I have included the text “Welcome to the Weather forecasting app.” whenever the isLoading state is false.
- As you can see, the isLoading state is associated with the data fetching process.
- Now with an example of code syntax let's see what happens when it is true.
export default class App extends React.Component {
state = {
isLoading: true
};
render() {
const { isLoading } = this.state;
return (
<View style={styles.container}>
{isLoading ? (
<Text>Please wait, while it is collecting the weather-based data.</Text>
) : (
<View>
- Here, I have changed the isLoading state to true. With this, I have rendered the text “Please wait, while it is collecting the weather-based data.” on the screen as the data is a loading screen, the user will see this text on their app screen.
Getting started with the weather-based component
Now, we will start designing the first screen of the app. In the title, you want to include the temperature and an icon. Also, I want to display text on this first screen. For this, follow the given below steps.
- Create a ./Component folder in your project directory. And then create a weather.js file in the component folder. Now start designing the first screen by adding different weather components.
- Under the parent Weather container, integrated two children containers: bodyContainer and titleContainer.
- For the icon on the title, get the third-party package @expo/vector-icons integrated with your project. Here, I have selected the Feather icon from the library. This is one of the advantages for which I selected the Expo tool.
- Below is the code snippet that you need to add to the weather.js file of the app’s root directory.
import React from "react";
import { View, Text, StyleSheet } from "react-native";
import { Feather } from "@expo/vector-icons";
const Weather = () => {
return (
<View style={styles.weatherContainer}>
<View style={styles.titleContainer}>
<Feather name="cloud-snow" size={58} color="#00ffff" />
<Text style={styles.tempText}>Live temperature </Text>
</View>
<View style={styles.bodyContainer}>
<Text style={styles.title}>So Chilled outside!!</Text>
<Text style={styles.subtitle}>My hands are getting cold.</Text>
</View>
</View>
);
};
const styles = StyleSheet.create({
weatherContainer: {
flex: 1,
backgroundColor: "#f8f8ff",
},
titleContainer: {
flex: 2,
alignItems: "center",
justifyContent: "center",
},
tempText: {
fontSize: 40,
color: "#00ffff",
},
bodyContainer: {
flex: 1,
alignItems: "flex-start",
justifyContent: "flex-end",
paddingLeft: 25,
marginBottom: 40,
},
title: {
fontSize: 40,
color: "#00ffff",
},
subtitle: {
fontSize: 26,
color: "#00ffff",
},
});
export default Weather;
- Look at how I added the texts of the title and subtitle. I also imported the Feather icon from the @expo/vector-icons. Refer to the image of the output given in image 2.
Image 2
Utility of API calls - weather forecasting
For weather-related data, you have to use the GET request and API calling method. This will further need to generate a specific API key. I will show you an easy method of getting a weather-based API key for your project. Click on the ‘API’ section and copy the API key.
A complete code snippet added to the app.js
Take reference to the below-given code syntax of how you can build a live weather forecasting app.
import React, { useState, useEffect } from "react";
import { Platform, Text, View, StyleSheet } from "react-native";
import * as Location from "expo-location";
const API_KEY = "5bd2e0405c5b05e7f1dcda04a58619b5";
export default function App() {
const [location, setLocation] = useState(null);
const [weatherData, setWeatherData] = useState(null);
const [loaded, setLoaded] = useState(true);
useEffect(() => {
const DataLocation = async () => {
let { status } = await Location.requestForegroundPermissionsAsync();
if (status !== "granted") {
setErrorMsg("Permission to access location was denied");
return;
}
let location = await Location.getCurrentPositionAsync({});
setLocation(location);
console.log(location, "tanusree");
};
DataLocation();
}, []);
async function fectWeatherData(location) {
let lat = location.coords.latitude;
let long = location.coords.longitude;
console.log(lat, long);
setLoaded(false);
const API = `https://api.openweathermap.org/data/2.5/weather?lat=${lat}&lon=${long}&appid=${API_KEY}&units=metric`;
console.log(API);
try {
const response = await fetch(API);
if (response.status == 200) {
const data = await response.json();
console.log(data, "12");
setWeatherData(data);
} else {
setWeatherData(null);
}
setLoaded(true);
} catch (error) {
console.log(error);
}
}
useEffect(() => {
fectWeatherData(location);
console.log(weatherData, "345");
}, [location]);
return (
<View style={styles.weatherContainer}>
<View style={styles.headerContainer}>
<Text style={styles.tempText}>
{weatherData && weatherData.main.temp}˚
</Text>
</View>
<View
style={{
flex: 1,
justifyContent: "flex-end",
marginLeft: 30,
marginBottom: 40,
}}
>
<Text style={{ fontSize: 40, color: "#FFFFFF" }}>
{weatherData && weatherData.weather[0].main}
</Text>
<Text style={{ fontSize: 20, color: "#FFFFFF" }}>
{weatherData && weatherData.weather[0].description}
</Text>
</View>
</View>
);
}
const styles = StyleSheet.create({
weatherContainer: {
flex: 1,
backgroundColor: "orange",
},
headerContainer: {
flexDirection: "row",
marginTop: 100,
justifyContent: "space-around",
},
tempText: {
fontSize: 72,
color: "#FFFFFF",
},
});
Get an output on your emulator screen as given in image 3.
Image 3
Summary
Building a weather app using React Native may need a few steps but what you need to learn is to add the required React Native components at the correct place in your codebase. You should also have a strong understanding of the utility of the react Native libraries. This can make your life less challenging as a developer. Go through this article and try now to learn about creating a weather forecasting app.