WordPress creating custom post types, meta box and taxonomy code example
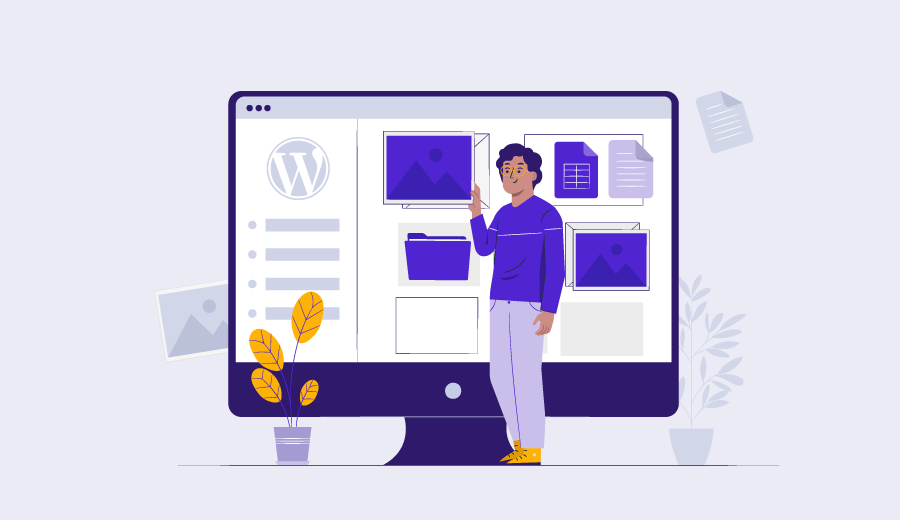
WordPress comes with a number of different post types already installed, including Post, Page, Revision, and Attachment. In a limited sense, they are an adequate representation of the subject of your blog. On the other hand, once your material takes on a more varied form, they could no longer be enough. The custom post type in WordPress comes into play at this point in the process. In a nutshell, it is an additional post type that you develop according to the requirements of your site. You'll be able to create more granular categories for your content with its help. When using WordPress, it is more time-efficient to create a custom post type than than apply categories to your individual posts. The reason for this is because organising articles into categories places them all on the same list, most frequently in the Post section. The difficulty is that if you have more than one general topic, it will be tough for you to maintain track of all of them in your head at the same time. On the other side, it gives you the ability to select a part that is more appropriate for your post right from the beginning. This should make it easier and more seamless for you to categorise the stuff you have. Every custom post type in WordPress has its own menu in the administration area of WordPress, and clicking on that menu takes you to a list of all of the relevant posts.
if( ! function_exists( 'create_quote_post_type' ) ): function create_quote_post_type() { $labels = array( 'name' = > __( 'Quote' ), 'singular_name' = > __( 'Quote' ), 'add_new' = > __( 'Add quote' ), 'all_items' = > __( 'All quotes' ), 'add_new_item' = > __( 'Add quote' ), 'edit_item' = > __( 'Edit quote' ), 'new_item' = > __( 'New quote' ), 'view_item' = > __( 'View quote' ), 'search_items' = > __( 'Search quotes' ), 'not_found' = > __( 'No quotes found' ), 'not_found_in_trash' = > __( 'No quotes found in trash' ), 'parent_item_colon' = > __( 'Parent quote' ) //'menu_name' = > default to 'name' ); $args = array( 'labels' = > $labels, 'public' = > true, 'publicly_queryable' = > true, 'query_var' = > true, 'rewrite' = > true, 'capability_type' = > 'post', 'hierarchical' = > false, 'supports' = > array( 'title', 'thumbnail', 'editor', 'author', 'excerpt', //'trackbacks', //'custom-fields', //'comments', 'revisions', //'page-attributes', // (menu order, hierarchical must be true to show Parent option) //'post-formats', ), 'taxonomies' = > array( 'category', 'post_tag' ), 'menu_position' = > 5, 'register_meta_box_cb' = > 'add_quote_post_type_metabox' ); register_post_type( 'quote', $args ); //flush_rewrite_rules(); register_taxonomy( 'custom_category', // register custom taxonomy 'quote', array( 'hierarchical' = > true, 'label' = > 'Custom category' ) ); } add_action( 'init', 'create_quote_post_type' ); endif; function add_quote_post_type_metabox() { // add the meta box add_meta_box( 'quote_metabox', 'Meta', 'quote_metabox', 'quote', 'normal' ); } function quote_metabox() { global $post; // Noncename needed to verify where the data originated echo ' <input name="quote_post_noncename" id="quote_post_noncename" value="' . wp_create_nonce( plugin_basename(__FILE__) ) . '" type="hidden" >'; // Get the data if its already been entered $quote_post_name = get_post_meta($post- >ID, '_quote_post_name', true); $quote_post_desc = get_post_meta($post- >ID, '_quote_post_desc', true); // Echo out the field ? > < div class="width_full p_box" > < p > <label >Name < input name="quote_post_name" class="widefat" value=" <?php echo $quote_post_name; ? >" type="text" > </label > </p > <p > <label >Description < textarea name="quote_post_desc" class="widefat" > <?php echo $quote_post_desc; ? > </textarea > </label > </p > </div > <?php } function quote_post_save_meta( $post_id, $post ) { // save the data // verify this came from the our screen and with proper authorization, // because save_post can be triggered at other times if( !wp_verify_nonce( $_POST['quote_post_noncename'], plugin_basename(__FILE__) ) ) { return $post- >ID; } // is the user allowed to edit the post or page? if( ! current_user_can( 'edit_post', $post- >ID )){ return $post- >ID; } // ok, we're authenticated: we need to find and save the data // we'll put it into an array to make it easier to loop though $quote_post_meta['_quote_post_name'] = $_POST['quote_post_name']; $quote_post_meta['_quote_post_desc'] = $_POST['quote_post_desc']; // add values as custom fields foreach( $quote_post_meta as $key = > $value ) { // cycle through the $quote_post_meta array // if( $post- >post_type == 'revision' ) return; // don't store custom data twice $value = implode(',', (array)$value); // if $value is an array, make it a CSV (unlikely) if( get_post_meta( $post- >ID, $key, FALSE ) ) { // if the custom field already has a value update_post_meta($post- >ID, $key, $value); } else { // if the custom field doesn't have a value add_post_meta( $post- >ID, $key, $value ); } if( !$value ) { // delete if blank delete_post_meta( $post- >ID, $key ); } } } add_action( 'save_post', 'quote_post_save_meta', 1, 2 ); // save the custom fields if( ! function_exists( 'view_quotes_posts' ) ): // output function view_quotes_posts( $num = 4, $do_shortcode = 1 ) { $args = array( 'numberposts' = > $num, 'offset' = > 0, //'category' = > , 'orderby' = > 'menu_order, post_title', // post_date, rand 'order' = > 'DESC', //'include' = > , //'exclude' = > , //'meta_key' = > , //'meta_value' = > , 'post_type' = > 'quote', //'post_mime_type' = > , //'post_parent' = > , 'post_status' = > 'publish', 'suppress_filters' = > true ); $posts = get_posts( $args ); $html = ''; foreach ( $posts as $post ) { $meta_name = get_post_meta( $post- >ID, '_quote_post_name', true ); $meta_desc = get_post_meta( $post- >ID, '_quote_post_desc', true ); $img = get_the_post_thumbnail( $post- >ID, 'medium' ); if( empty( $img ) ) { $img = ' <img src="'.plugins_url( '/img/default.png', __FILE__ ).'" >'; } if( has_post_thumbnail( $post- >ID ) ) { $image = wp_get_attachment_image_src( get_post_thumbnail_id( $post- >ID ), 'single-post-thumbnail' ); $img_url = $image[0]; //the_post_thumbnail( 'thumbnail' ); /* thumbnail, medium, large, full, thumb-100, thumb-200, thumb-400, array(100,100) */ } //$content = $post- >post_content; if( $do_shortcode == 1) { $content = do_shortcode( $post- >post_content ); }else{ $content = strip_shortcodes( $post- >post_content ); } $html .= ' <div > <h3 >'.$post- >post_title.' </h3 > <div > <p >Name: '.$meta_name.' </p > <p >Description: '.$meta_desc.' </p > </div > <div >'.$img.' </div > <div >'.$content.' </div > </div > '; } $html = ' <div class="wrapper" >'.$html.' </div >'; return $html; } endif;